Rails Secret_key_base Generate
Have you ever wondered about those secret keys found in config/secrets.yml
of your Rails app? The comments generated in that file describe the keys as such:
- Rails Secret_key_base Generate Table
- Rails Generate Secret Key Base
- Rails Secret_key_base Generate System
- Rails Generate Secret_key_base For Production
- Rails Generate Secret_key_base
Nowadays (rails 6) rails generate a secret key base in tmp/developmentsecret.txt for you. And in production environment the best is having SECRETKEYBASE as en env variable, it will get picked up by rails. You can check with Rails.application.secretkeybase. Use your existing secretkeybase from the secrettoken.rb initializer to set the SECRETKEYBASE environment variable for whichever users running the Rails application in production mode. Alternatively, you can simply copy the existing secretkeybase from the secrettoken.rb initializer to secrets.yml under the production section, replacing '<%= ENV'
‘Your secret key is used for verifying the integrity of signed cookies.’
Great… but what if they become compromised? Or we need to change them? We can generate new ones.
Rails provides rake secret
for just this purpose.
The source code is here. The code simply requires SecureRandom
and spits out a string. If you want to be really clever, you can pipe the string directly into your Vim buffer for the config file, with :.! rake secret
.
Check out rake -T secret
inside a Rails root directory for more information.
1 Upgrading to Rails 4.1
If you're upgrading an existing application, it's a great idea to have good testcoverage before going in. You should also first upgrade to Rails 4.0 in case youhaven't and make sure your application still runs as expected before attemptingan update to Rails 4.1. A list of things to watch out for when upgrading isavailable in theUpgrading Ruby on Railsguide.
2 Major Features
2.1 Spring Application Preloader
Spring is a Rails application preloader. It speeds up development by keepingyour application running in the background so you don't need to boot it everytime you run a test, rake task or migration.
New Rails 4.1 applications will ship with 'springified' binstubs. This meansthat bin/rails
and bin/rake
will automatically take advantage of preloadedspring environments.
Running rake tasks:
Running a Rails command:
Spring introspection:
Have a look at theSpring README tosee all available features.
See the Upgrading Ruby on Railsguide on how to migrate existing applications to use this feature.
2.2 config/secrets.yml
Rails 4.1 generates a new secrets.yml
file in the config
folder. By default,this file contains the application's secret_key_base
, but it could also beused to store other secrets such as access keys for external APIs.
The secrets added to this file are accessible via Rails.application.secrets
.For example, with the following config/secrets.yml
:
Rails.application.secrets.some_api_key
returns SOMEKEY
in the developmentenvironment.
See the Upgrading Ruby on Railsguide on how to migrate existing applications to use this feature.
2.3 Action Pack Variants
We often want to render different HTML/JSON/XML templates for phones,tablets, and desktop browsers. Variants make it easy.
The request variant is a specialization of the request format, like :tablet
,:phone
, or :desktop
.
Rails Secret_key_base Generate Table
You can set the variant in a before_action
:
Respond to variants in the action just like you respond to formats:
Provide separate templates for each format and variant:
You can also simplify the variants definition using the inline syntax:
2.4 Action Mailer Previews
Action Mailer previews provide a way to see how emails look by visitinga special URL that renders them.
You implement a preview class whose methods return the mail object you'd liketo check:
The preview is available in http://localhost:3000/rails/mailers/notifier/welcome,and a list of them in http://localhost:3000/rails/mailers.
By default, these preview classes live in test/mailers/previews
.This can be configured using the preview_path
option.
See itsdocumentationfor a detailed write up.
2.5 Active Record enums
Declare an enum attribute where the values map to integers in the database, butcan be queried by name.
See itsdocumentationfor a detailed write up.
2.6 Message Verifiers
Message verifiers can be used to generate and verify signed messages. This canbe useful to safely transport sensitive data like remember-me tokens andfriends.
The method Rails.application.message_verifier
returns a new message verifierthat signs messages with a key derived from secret_key_base and the givenmessage verifier name:
2.7 Module#concerning
A natural, low-ceremony way to separate responsibilities within a class:
This example is equivalent to defining a EventTracking
module inline,extending it with ActiveSupport::Concern
, then mixing it in to theTodo
class.
See itsdocumentationfor a detailed write up and the intended use cases.
2.8 CSRF protection from remote <script>
tags
Cross-site request forgery (CSRF) protection now covers GET requests withJavaScript responses, too. That prevents a third-party site from referencingyour JavaScript URL and attempting to run it to extract sensitive data.
Rails Generate Secret Key Base
This means any of your tests that hit .js
URLs will now fail CSRF protectionunless they use xhr
. Upgrade your tests to be explicit about expectingXmlHttpRequests. Instead of post :create, format: :js
, switch to the explicitxhr :post, :create, format: :js
.
3 Railties
Please refer to theChangelogfor detailed changes.
3.1 Removals
Removed
update:application_controller
rake task.Removed deprecated
Rails.application.railties.engines
.Removed deprecated
threadsafe!
from Rails Config.Removed deprecated
ActiveRecord::Generators::ActiveModel#update_attributes
infavor ofActiveRecord::Generators::ActiveModel#update
.Removed deprecated
config.whiny_nils
option.Removed deprecated rake tasks for running tests:
rake test:uncommitted
andrake test:recent
.
3.2 Notable changes
The Spring applicationpreloader is now installedby default for new applications. It uses the development group ofthe
Gemfile
, so will not be installed inproduction. (Pull Request)BACKTRACE
environment variable to show unfiltered backtraces for testfailures. (Commit)Exposed
MiddlewareStack#unshift
to environmentconfiguration. (Pull Request)Added
Application#message_verifier
method to return a messageverifier. (Pull Request)The
test_help.rb
file which is required by the default generated testhelper will automatically keep your test database up-to-date withdb/schema.rb
(ordb/structure.sql
). It raises an error ifreloading the schema does not resolve all pending migrations. Opt outwithconfig.active_record.maintain_test_schema = false
. (PullRequest)Introduce
Rails.gem_version
as a convenience method to returnGem::Version.new(Rails.version)
, suggesting a more reliable way to performversion comparison. (Pull Request)
4 Action Pack
Please refer to theChangelogfor detailed changes.
4.1 Removals
Removed deprecated Rails application fallback for integration testing, set
ActionDispatch.test_app
instead.Removed deprecated
page_cache_extension
config.Removed deprecated
ActionController::RecordIdentifier
, useActionView::RecordIdentifier
instead.Removed deprecated constants from Action Controller:

Removed | Successor |
---|---|
ActionController::AbstractRequest | ActionDispatch::Request |
ActionController::Request | ActionDispatch::Request |
ActionController::AbstractResponse | ActionDispatch::Response |
ActionController::Response | ActionDispatch::Response |
ActionController::Routing | ActionDispatch::Routing |
ActionController::Integration | ActionDispatch::Integration |
ActionController::IntegrationTest | ActionDispatch::IntegrationTest |
4.2 Notable changes
protect_from_forgery
also prevents cross-origin<script>
tags.Update your tests to usexhr :get, :foo, format: :js
instead ofget :foo, format: :js
.(Pull Request)#url_for
takes a hash with options inside anarray. (Pull Request)Added
session#fetch
method fetch behaves similarly toHash#fetch,with the exception that the returned value is always saved into thesession. (Pull Request)Separated Action View completely from ActionPack. (Pull Request)
Log which keys were affected by deepmunge. (Pull Request)
New config option
config.action_dispatch.perform_deep_munge
to opt out ofparams 'deep munging' that was used to address security vulnerabilityCVE-2013-0155. (Pull Request)New config option
config.action_dispatch.cookies_serializer
for specifying aserializer for the signed and encrypted cookie jars. (Pull Requests1,2 /More Details)Added
render :plain
,render :html
andrender:body
. (Pull Request /More Details)
5 Action Mailer
Please refer to theChangelogfor detailed changes.
5.1 Notable changes
Added mailer previews feature based on 37 Signals mail_viewgem. (Commit)
Instrument the generation of Action Mailer messages. The time it takes togenerate a message is written to the log. (Pull Request)
6 Active Record
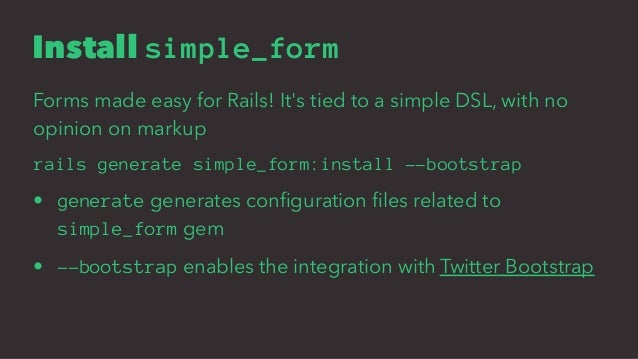
Please refer to theChangelogfor detailed changes.
6.1 Removals
Removed deprecated nil-passing to the following
SchemaCache
methods:primary_keys
,tables
,columns
andcolumns_hash
.Removed deprecated block filter from
ActiveRecord::Migrator#migrate
.Removed deprecated String constructor from
ActiveRecord::Migrator
.Removed deprecated
scope
use without passing a callable object.Removed deprecated
transaction_joinable=
in favor ofbegin_transaction
with a:joinable
option.Removed deprecated
decrement_open_transactions
.Removed deprecated
increment_open_transactions
.Removed deprecated
PostgreSQLAdapter#outside_transaction?
method. You can use#transaction_open?
instead.Removed deprecated
ActiveRecord::Fixtures.find_table_name
in favor ofActiveRecord::Fixtures.default_fixture_model_name
.Removed deprecated
columns_for_remove
fromSchemaStatements
.Removed deprecated
SchemaStatements#distinct
.Moved deprecated
ActiveRecord::TestCase
into the Rails testsuite. The class is no longer public and is only used for internalRails tests.Removed support for deprecated option
:restrict
for:dependent
in associations.Removed support for deprecated
:delete_sql
,:insert_sql
,:finder_sql
and:counter_sql
options in associations.Removed deprecated method
type_cast_code
from Column.Removed deprecated
ActiveRecord::Base#connection
method.Make sure to access it via the class.Removed deprecation warning for
auto_explain_threshold_in_seconds
.Removed deprecated
:distinct
option fromRelation#count
.Removed deprecated methods
partial_updates
,partial_updates?
andpartial_updates=
.Removed deprecated method
scoped
.Removed deprecated method
default_scopes?
.Remove implicit join references that were deprecated in 4.0.
Removed
activerecord-deprecated_finders
as a dependency.Please see the gem READMEfor more info.Removed usage of
implicit_readonly
. Please usereadonly
methodexplicitly to mark records asreadonly
. (Pull Request)
6.2 Deprecations
Deprecated
quoted_locking_column
method, which isn't used anywhere.Deprecated
ConnectionAdapters::SchemaStatements#distinct
,as it is no longer used by internals. (Pull Request)Deprecated
rake db:test:*
tasks as the test database is nowautomatically maintained. See railties release notes. (PullRequest)Deprecate unused
ActiveRecord::Base.symbolized_base_class
andActiveRecord::Base.symbolized_sti_name
withoutreplacement. Commit
6.3 Notable changes
- Default scopes are no longer overridden by chained conditions.
Before this change when you defined a default_scope
in a model it was overridden by chained conditions in the same field. Now it is merged like any other scope. More Details.
Added
ActiveRecord::Base.to_param
for convenient 'pretty' URLs derived froma model's attribute ormethod. (Pull Request)Added
ActiveRecord::Base.no_touching
, which allows ignoring touch onmodels. (Pull Request)Unify boolean type casting for
MysqlAdapter
andMysql2Adapter
.type_cast
will return1
fortrue
and0
forfalse
. (Pull Request).unscope
now removes conditions specified indefault_scope
. (Commit)Added
ActiveRecord::QueryMethods#rewhere
which will overwrite an existing,named where condition. (Commit)Extended
ActiveRecord::Base#cache_key
to take an optional list of timestampattributes of which the highest will be used. (Commit)Added
ActiveRecord::Base#enum
for declaring enum attributes where the valuesmap to integers in the database, but can be queried byname. (Commit)Type cast json values on write, so that the value is consistent with readingfrom the database. (Pull Request)
Type cast hstore values on write, so that the value is consistentwith reading from the database. (Commit)
Make
next_migration_number
accessible for third partygenerators. (Pull Request)Calling
update_attributes
will now throw anArgumentError
whenever itgets anil
argument. More specifically, it will throw an error if theargument that it gets passed does not respond to tostringify_keys
. (Pull Request)CollectionAssociation#first
/#last
(e.g.has_many
) use aLIMIT
edquery to fetch results rather than loading the entirecollection. (Pull Request)inspect
on Active Record model classes does not initiate a newconnection. This means that callinginspect
, when the database is missing,will no longer raise an exception. (Pull Request)Removed column restrictions for
count
, let the database raise if the SQL isinvalid. (Pull Request)Rails now automatically detects inverse associations. If you do not set the
:inverse_of
option on the association, then Active Record will guess theinverse association based on heuristics. (Pull Request)Handle aliased attributes in ActiveRecord::Relation. When using symbol keys,ActiveRecord will now translate aliased attribute names to the actual columnname used in the database. (Pull Request)
The ERB in fixture files is no longer evaluated in the context of the mainobject. Helper methods used by multiple fixtures should be defined on modulesincluded in
ActiveRecord::FixtureSet.context_class
. (Pull Request)Don't create or drop the test database if RAILS_ENV is specifiedexplicitly. (Pull Request)
Relation
no longer has mutator methods like#map!
and#delete_if
. Convertto anArray
by calling#to_a
before using these methods. (Pull Request)find_in_batches
,find_each
,Result#each
andEnumerable#index_by
nowreturn anEnumerator
that can calculate itssize. (Pull Request)scope
,enum
and Associations now raise on 'dangerous' nameconflicts. (Pull Request,Pull Request)second
throughfifth
methods act like thefirst
finder. (Pull Request)Make
touch
fire theafter_commit
andafter_rollback
callbacks. (Pull Request)Enable partial indexes for
sqlite >= 3.8.0
.(Pull Request)Make
change_column_null
revertible. (Commit)Added a flag to disable schema dump after migration. This is set to
false
by default in the production environment for new applications.(Pull Request)
7 Active Model
Please refer to theChangelogfor detailed changes.
7.1 Deprecations
- Deprecate
Validator#setup
. This should be done manually now in thevalidator's constructor. (Commit)
7.2 Notable changes
Added new API methods
reset_changes
andchanges_applied
toActiveModel::Dirty
that control changes state.Ability to specify multiple contexts when defining avalidation. (Pull Request)
attribute_changed?
now accepts a hash to check if the attribute was changed:from
and/or:to
a givenvalue. (Pull Request)
8 Active Support
Please refer to theChangelogfor detailed changes.
Rails Secret_key_base Generate System
8.1 Removals
Removed
MultiJSON
dependency. As a result,ActiveSupport::JSON.decode
no longer accepts an options hash forMultiJSON
. (Pull Request / More Details)Removed support for the
encode_json
hook used for encoding custom objects intoJSON. This feature has been extracted into the activesupport-json_encodergem.(Related Pull Request /More Details)Removed deprecated
ActiveSupport::JSON::Variable
with no replacement.Removed deprecated
String#encoding_aware?
core extensions (core_ext/string/encoding
).Removed deprecated
Module#local_constant_names
in favor ofModule#local_constants
.Removed deprecated
DateTime.local_offset
in favor ofDateTime.civil_from_format
.Removed deprecated
Logger
core extensions (core_ext/logger.rb
).Removed deprecated
Time#time_with_datetime_fallback
,Time#utc_time
andTime#local_time
in favor ofTime#utc
andTime#local
.Removed deprecated
Hash#diff
with no replacement.Removed deprecated
Date#to_time_in_current_zone
in favor ofDate#in_time_zone
.Removed deprecated
Proc#bind
with no replacement.Removed deprecated
Array#uniq_by
andArray#uniq_by!
, use nativeArray#uniq
andArray#uniq!
instead.Removed deprecated
ActiveSupport::BasicObject
, useActiveSupport::ProxyObject
instead.Removed deprecated
BufferedLogger
, useActiveSupport::Logger
instead.Removed deprecated
assert_present
andassert_blank
methods, useassertobject.blank?
andassert object.present?
instead.Remove deprecated
#filter
method for filter objects, use the correspondingmethod instead (e.g.#before
for a before filter).Removed 'cow' => 'kine' irregular inflection from defaultinflections. (Commit)
8.2 Deprecations
Deprecated
Numeric#{ago,until,since,from_now}
, the user is expected toexplicitly convert the value into an AS::Duration, i.e.5.ago
=>5.seconds.ago
(Pull Request)Deprecated the require path
active_support/core_ext/object/to_json
. Requireactive_support/core_ext/object/json
instead. (Pull Request)Deprecated
ActiveSupport::JSON::Encoding::CircularReferenceError
. This featurehas been extracted into the activesupport-json_encodergem.(Pull Request /More Details)Deprecated
ActiveSupport.encode_big_decimal_as_string
option. This feature hasbeen extracted into the activesupport-json_encodergem.(Pull Request /More Details)Deprecate custom
BigDecimal
serialization. (Pull Request)
8.3 Notable changes
ActiveSupport
's JSON encoder has been rewritten to take advantage of theJSON gem rather than doing custom encoding in pure-Ruby.(Pull Request /More Details)Improved compatibility with the JSON gem.(Pull Request /More Details)
Added
ActiveSupport::Testing::TimeHelpers#travel
and#travel_to
. Thesemethods change current time to the given time or duration by stubbingTime.now
andDate.today
.Added
ActiveSupport::Testing::TimeHelpers#travel_back
. This method returnsthe current time to the original state, by removing the stubs added bytravel
andtravel_to
. (Pull Request)Added
Numeric#in_milliseconds
, like1.hour.in_milliseconds
, so we can feedthem to JavaScript functions likegetTime()
. (Commit)Added
Date#middle_of_day
,DateTime#middle_of_day
andTime#middle_of_day
methods. Also addedmidday
,noon
,at_midday
,at_noon
andat_middle_of_day
asaliases. (Pull Request)Added
Date#all_week/month/quarter/year
for generating dateranges. (Pull Request)Added
Time.zone.yesterday
andTime.zone.tomorrow
. (Pull Request)Added
String#remove(pattern)
as a short-hand for the common pattern ofString#gsub(pattern,')
. (Commit)Added
Hash#compact
andHash#compact!
for removing items with nil valuefrom hash. (Pull Request)blank?
andpresent?
commit to returnsingletons. (Commit)Default the new
I18n.enforce_available_locales
config totrue
, meaningI18n
will make sure that all locales passed to it must be declared in theavailable_locales
list. (Pull Request)Introduce
Module#concerning
: a natural, low-ceremony way to separateresponsibilities within aclass. (Commit)Added
Object#presence_in
to simplify adding values to a permitted list.(Commit)
Rails Generate Secret_key_base For Production
9 Credits
See thefull list of contributors to Rails forthe many people who spent many hours making Rails, the stable and robustframework it is. Kudos to all of them.
Feedback
You're encouraged to help improve the quality of this guide.
Rails Generate Secret_key_base
Please contribute if you see any typos or factual errors. To get started, you can read our documentation contributions section.
You may also find incomplete content or stuff that is not up to date. Please do add any missing documentation for master. Make sure to check Edge Guides first to verify if the issues are already fixed or not on the master branch. Check the Ruby on Rails Guides Guidelines for style and conventions.
If for whatever reason you spot something to fix but cannot patch it yourself, please open an issue.
And last but not least, any kind of discussion regarding Ruby on Rails documentation is very welcome on the rubyonrails-docs mailing list.